452. Minimum Number of Arrows to Burst Balloons
Description
There are some spherical balloons taped onto a flat wall that represents the
XY-plane. The balloons are represented as a 2D integer array points
where
points[i] = [xstart, xend]
denotes a balloon whose horizontal diameter
stretches between xstart
and xend
. You do not know the exact y-coordinates
of the balloons.
Arrows can be shot up directly vertically (in the positive y-direction) from different points along the x-axis. A balloon with xstart
and xend
is burst by an arrow shot at x if xstart <= x <= xend
. There is no limit to the number of arrows that can be shot. A shot arrow keeps traveling up infinitely, bursting any balloons in its path.
Given the array points
, return the minimum number of arrows that must be
shot to burst all balloons.
Example
points = [[10,16],[2,8],[1,6],[7,12]]
- Shoot an arrow at x = 6, bursting the balloons [2,8] and [1,6].
- Shoot an arrow at x = 11, bursting the balloons [10,16] and [7,12]
The balloons can be burst by 2 arrows:
Intuition
- There are several
segments
on the one-dimensional coordinate axis, find out theminimum
number of arrows which are orthogonal to the coordinate axis so that each segment can be shot through by at least onearrow
.
Approaches
Using Sorting
-
Given points
-
Sort the segments by the end
sort(points.begin(), points.end(), [&](auto &x, auto &y) { return x[1] < y[1]; });
-
Put an arrow at the end of the
1-st
segment -
From the
2-nd
segment, we check whether the current arrow pass through the currentsegment
, if not add an arrow, put it at the end of the current segment
Time Complexity
O(N * log(N))
where 'N' is points length
Space Complexity
O(1)
Code
class Solution {
public:
int findMinArrowShots(vector<vector<int>>& points) {
sort(points.begin(), points.end(), [&](auto &x, auto &y) {
return x[1] < y[1];
});
int n = points.size(), ans = 1, max = points[0][1];
for(int i = 1; i < n; i++) {
if(points[i][0] > max) {
ans++;
max = points[i][1];
}
}
return ans;
}
};
Analysis
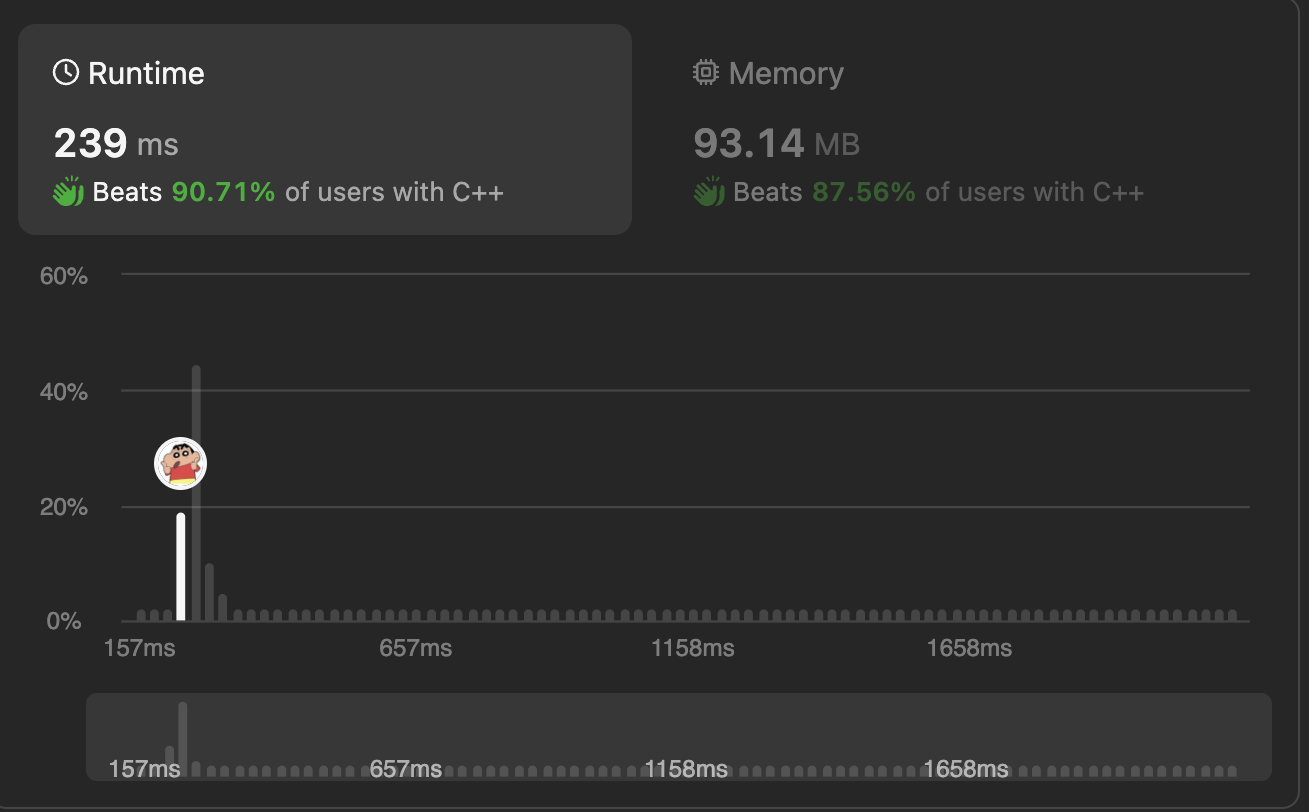